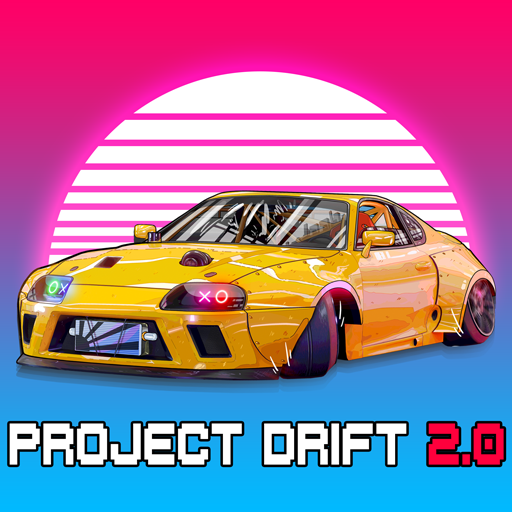
Project Drift
A downloadable game for Windows, macOS, Linux, and Android
Download NowName your own price
drift game
Status | In development |
Platforms | Windows, macOS, Linux, Android |
Author | GoEatToothPaste |
Download
Download NowName your own price
Click download now to get access to the following files:
DriftHunters_v1.2_PC.zip 145 MB
Comments
Log in with itch.io to leave a comment.
using UnityEngine;
public class PunchingSystem : MonoBehaviour
{
public Collider punchCollider;
public float punchCooldown = 1f;
private float nextPunchTime = 0f;
void Update()
{
if (Input.GetKeyDown(KeyCode.Space) && Time.time >= nextPunchTime)
{
// Trigger punch animation if you have one
GetComponent<Animator>().SetTrigger("Punch");
// Enable the punch collider
punchCollider.enabled = true;
// Set the cooldown for the next punch
nextPunchTime = Time.time + punchCooldown;
}
}
void OnTriggerEnter(Collider other)
{
// Check if the punching collider hits the target
if (other.CompareTag("Target"))
{
Debug.Log("Target hit!");
// Handle the target hit, such as reducing health or triggering a specific effect
}
}
void OnTriggerExit(Collider other)
{
// Disable the punch collider after the punch
punchCollider.enabled = false;
}
}
using System.Collections;
using UnityEngine;
public class SimpleWalker : MonoBehaviour
{
private CharacterController characterController;
public float speed = 5f;
void Start()
{
characterController = GetComponent<CharacterController>();
}
void Update()
{
float horizontal = Input.GetAxis("Horizontal");
float vertical = Input.GetAxis("Vertical");
Vector3 direction = new Vector3(horizontal, 0f, vertical).normalized;
Vector3 move = direction * speed * Time.deltaTime;
characterController.Move(move);
}
}
this game in in alpha